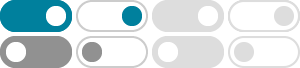
Reading c file line by line using fgetc() - Stack Overflow
2010年11月27日 · If you are not going to use fgets() (perhaps because you want to remove the newline, or you want to deal with "\r", "\n" or "\r\n" line endings, or you want to know how many characters were read), you can use this as a skeleton function:
c - getc () vs fgetc () - What are the major differences ... - Stack ...
2013年8月28日 · The difference between getc and fgetc is that getc can be implemented as a macro, whereas fgetc cannot be implemented as a macro. This means three things: The argument to getc should not be an expression with side effects. Since fgetc is guaranteed to be a function, we can take its address.
c - fgetc (): Is it enough to just check EOF? - Stack Overflow
the fgetc() function shall obtain the next byte as an unsigned char converted to an int The following macro name shall be defined as a negative integer constant expression: EOF As long as you store the return value in an int and not a char , it is sufficient to check for EOF because it is guaranteed not to represent a valid character value.
c - fgetc, checking EOF - Stack Overflow
If type char is signed, and if EOF is defined (as is usual) as -1, the character with the decimal value 255 ('\377' or '\xff' in C) will be sign-extended and will compare equal to EOF, prematurely terminating the input.
c - difference between the fgetc() and fgets()? - Stack Overflow
2014年3月5日 · The API could have been made another way, for example fgetc() could return char*, NULL for EOF and otherwise a pointer to an internal (static like strtok!) array of two chars: the one from the stream followed by a \0 for convenience. Then the two functions would return the same thing (a C string), and use the same sentinel value.
c - What are all the reasons `fgetc ()` might return `EOF`? - Stack ...
2022年1月6日 · The C library lists three cases where fgetc() returns EOF. If the end-of-file indicator for the stream is set, or if the stream is at end-of-file, the end-of-file indicator for the stream is set and the fgetc function returns EOF. Otherwise, the fgetc function returns the
c - Exact functionality of fgetc & fread unclear - Stack Overflow
2025年3月4日 · A “character” in C is a single byte that fits in a char or unsigned char object. This design of C antedates wide use of multibyte character sets. Multibyte characters are different objects in C and are not supported by fgetc; fgetc is for reading raw bytes, not general characters with multibyte encodings.
c - What is the better way to check EOF and error of fgetc()?
2015年9月18日 · I always use this approach. int c; while ((c = fgetc(fp))!=EOF) { printf("%c", c); } As it seems to me more readable and robust.
Best way to portably assign the result of fgetc() to a char in C
2013年10月8日 · You are absolutely correct about the nature of fgetc()'s return value, but that wasn't my question. The crux is that converting an integer value to a signed integer type produces implementation-defined behavior if the value is outside the range of the target type (or please correct me if I'm wrong).
Fastest fgets implementation in C - Stack Overflow
2018年7月7日 · The known fgets libc function implementation uses fgetc() inside, how can I use read() with bigger buffer or other method instead to speed up the function? For example I read /proc/pid/maps file to search some strings. The file's format is known, currently I use the fgets implementation in the link with read(fd, &c, 1); instead of getc. I think ...