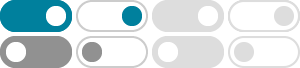
Which is the best way to get input from user in C?
2012年2月14日 · The scanf is the standard method to get formatted input in C, and fgets/fgetc is the recommended standard function to get whole lines or single characters. Most other functions are either non-standard or platform specific. –
How to read / parse input in C? The FAQ - Stack Overflow
2016年2月3日 · In plain ISO C, calling fflush() on an input stream has undefined behaviour. It does have well-defined behavior in POSIX and in MSVC, but neither of those make it discard user input that has not yet been read. Usually, the right way to clear pending input is read and discard characters up to and including a newline, but not beyond:
io - How to read a line from the console in C? - Stack Overflow
2008年11月24日 · There is a simple regex like syntax that can be used inside scanf to take whole line as input. scanf ...
How do I read a string entered by the user in C?
Here's a little snippet I use for line input from the user: #include <stdio.h> #include <string.h> #define OK 0 #define NO_INPUT 1 #define TOO_LONG 2 static int getLine (char *prmpt, char *buff, size_t sz) { int ch, extra; // Get line with buffer overrun protection.
Passing an array as an argument to a function in C
2011年7月4日 · How to pass a multidimensional [C-style] array to a function in C and C++, and here: How to pass a multidimensional array to a function in C++ only, via std::vector<std::vector<int>>& Passing 1D arrays as function parameters in C (and C++) 1. Standard array usage in C with natural type decay (adjustment) from array to ptr
Taking continuous input from user in C - Stack Overflow
2014年11月6日 · Actually just got it didn't realize that the fgets puts a \n at the end of the input string so just add \n to the end of the strcmp line and it works perfect! – atg963 Commented Nov 6, 2014 at 4:27
How to input strings into an array in C? - Stack Overflow
2017年1月7日 · While you can use scanf you will quickly run into one of the many pitfalls with taking user input with scanf that plague all new C programmers (e.g. failing to handle the '\n' left in the input buffer, failing to handle whitespace in strings, failing to limit the number of characters read/written, failing to validate the read or handle EOF, etc...
Input validation in C - Stack Overflow
2012年10月21日 · I'm writing a program in C. In the program, the user has to choose a number, either 1, 2 or 3. I would like to construct the code so that when the user enters in a number that isn't 1, 2 or 3, he/she
io - How can I clear an input buffer in C? - Stack Overflow
The C runtime library will take care of that for you: your program will see just '\n' for newlines. If you typed a character and pressed enter, then that input character would be read by line 1, and then '\n' would be read by line 2. See I'm using scanf %c to read a Y/N response, but later input gets skipped. from the comp.lang.c FAQ.
How to use a loop function with user input in C? - Stack Overflow
2013年4月27日 · One concern with this solution is that the scanf("%c", &c) is going to pick up the newline left in the input by the previous scanf("%f", &f3), and the newline is not going to be either N or n so the loop will continue (indeed, it won't wait for the user to type before continuing).