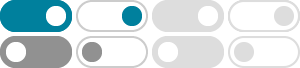
C++ sorting and keeping track of indexes - Stack Overflow
2009年10月16日 · You could sort std::pair instead of just ints - first int is original data, second int is original index. Then supply a comparator that only sorts on the first int. Example: Your problem instance: v = [5 7 8] New problem instance: v_prime = [<5,0>, <8,1>, <7,2>] Sort the new problem instance using a comparator like:
c++ - How to sort an STL vector? - Stack Overflow
2017年8月9日 · Like explained in other answers you need to provide a comparison function. If you would like to keep the definition of that function close to the sort call (e.g. if it only makes sense for this sort) you can define it right there with boost::lambda. Use boost::lambda::bind to call the member function. To e.g. sort by member variable or function ...
How to use std::sort to sort an array in C++ - Stack Overflow
2011年5月5日 · sort() can be applied on both array and vector in C++ to sort or re-arrange elements . 1. C++ sort() in case of a vector: // importing vector, algorithm & iostream. using namespace std; int main() {vector v = {5,4,3,2,8}; // depending on your vector size. sort(v.begin(), v.end()); cout<<v[1]; //testing the sorted element positions by printing ...
Sort based on multiple things in C++ - Stack Overflow
2010年8月26日 · This allows you to easy use of std::sort algorithm. Sorting itself will look like this: std::sort(X, X + 100, &CompareRecords); EDIT. You may even want to implement operator < for this structure -- in that case you can normally compare two objects of Record structure with operator <. And then you don't need to add the third parameter to std::sort.
c++ - Sorting a vector in descending order - Stack Overflow
2012年1月27日 · TL;DR. Use any. They are almost the same. Boring answer. As usual, there are pros and cons. Use std::reverse_iterator:
c++ - How do I sort a vector of custom objects? - Stack Overflow
2024年5月6日 · $ g++ -O2 -o sort sort.cpp && ./sort Here are results: Measure sort_by_operator on 10000000 items: Took 0 ...
c++ - Sorting std::map using value - Stack Overflow
2011年2月20日 · You can't sort a std::map this way, because a the entries in the map are sorted by the key. If you want to sort by value, you need to create a new std::map with swapped key and value. map<long, double> testMap; map<double, long> testMap2; // Insert values from testMap to testMap2 // The values in testMap2 are sorted by the double value
c++ - How to sort with a lambda? - Stack Overflow
2015年2月19日 · sort(mMyClassVector.begin(), mMyClassVector.end(), [](const MyClass & a, const MyClass & b) { return a.mProperty > b.mProperty; }); I'd like to use a lambda ...
What is the difference between std::sort and std::stable_sort?
2020年2月21日 · In the case of HP / Microsoft STL, std::stable_sort is a hybrid bottom up merge sort, using insertion sort to create sorted groups of 32 elements, then doing bottom up merge sort with the groups. The array (or vector) is split into two, a temporary array (or vector) 1/2 the size of the array to be sorted is allocated, and used to do a merge ...
c++ custom compare function for std::sort () - Stack Overflow
I want to create custom compare function for std::sort(), to sort some key-value pairs std::pair Here is my function template <typename K, typename V> int comparePairs(const void* left, c...