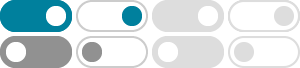
fstream - C++ Users
fstream Input/output stream class to operate on files. Objects of this class maintain a filebuf object as their internal stream buffer , which performs input/output operations on the file they are associated with (if any).
<fstream> - C++ Users
basic_fstream File stream (class template) basic_filebuf File stream buffer (class template) Classes Narrow characters (char) ifstream Input file stream class (class) ofstream Output file stream (class) fstream Input/output file stream class (class) filebuf File stream buffer (class) Wide characters (wchar_t)
Input/output with files - C++ Users
fstream: Stream class to both read and write from/to files. These classes are derived directly or indirectly from the classes istream and ostream . We have already used objects whose types were these classes: cin is an object of class istream and cout is an object of class ostream .
fstream - C++ Users
Constructs a fstream object, initially associated with the file identified by its first argument (filename), open with the mode specified by mode. Internally, its iostream base constructor is passed a pointer to a newly constructed filebuf object (the internal file stream buffer ).
fstream - C++ Users
// fstream::open / fstream::close #include <fstream> // std::fstream int main { std::fstream fs; fs.open ("test.txt", std::fstream::in | std::fstream::out | std::fstream::app); fs << " more lorem ipsum"; fs.close(); return 0; }
basic_ifstream - C++ Users
Template parameters charT Character type. This shall be a non-array POD type. Aliased as member type basic_ifstream::char_type. traits Character traits class that defines essential properties of the characters used by stream object (see char_traits). traits::char_type shall be the same as charT. Aliased as member type basic_ifstream::traits_type. Template instantiations
ofstream - C++ Users
Output stream class to operate on files. Objects of this class maintain a filebuf object as their internal stream buffer, which performs input/output operations on the file they are associated with (if any). File streams are associated with files either on construction, or by calling member open. This is an instantiation of basic_ofstream with the following template parameters:
ifstream - C++ Users
// ifstream constructor. #include <iostream> // std::cout #include <fstream> // std::ifstream int main { std::ifstream ifs ("test.txt", std::ifstream::in); char c = ifs.get(); while (ifs.good()) { std::cout << c; c = ifs.get(); } ifs.close(); return 0; }
basic_fstream - C++ Users
// fstream::open / fstream::close #include <fstream> // std::fstream int main { std::fstream fs; fs.open ("test.txt", std::fstream::in | std::fstream::out | std::fstream::app); fs << " more lorem ipsum"; fs.close(); return 0; }
filebuf - C++ Users
// filebuf::open() #include <iostream> #include <fstream> int main { std::ifstream is; std::filebuf * fb = is.rdbuf(); fb->open ("test.txt",std::ios::out|std::ios::app); // >> appending operations here << fb->close(); return 0; }