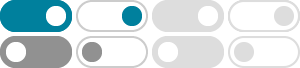
Best and/or fastest way to create lists in python
If you want to see the dependency with the length of the list n: Pure python. I tested for list length up to n=10000 and the behavior remains the same. So the integer multiplication method is the fastest with difference. Numpy. For lists with more than ~300 elements you should consider numpy. Benchmark code:
python - how to create a list of lists - Stack Overflow
2012年9月6日 · In order to create a list of lists you will have firstly to store your data, array, or other type of variable into a list. Then, create a new empty list and append to it the lists that you just created. At the end you should end up with a list of lists: list_1=data_1.tolist() list_2=data_2.tolist() listoflists = [] listoflists.append(list_1 ...
List of zeros in python - Stack Overflow
2011年12月16日 · There is a gotcha though, both itertools.repeat and [0] * n will create lists whose elements refer to same id. This is not a problem with immutable objects like integers or strings but if you try to create list of mutable objects like a list of lists ([[]] * n) then all the elements will refer to the same object.
python - How do I make a flat list out of a list of lists ... - Stack ...
2016年12月3日 · A list of lists named xss can be flattened using a nested list comprehension: flat_list = [ x for xs in xss for x in xs ] The above is equivalent to: flat_list = [] for xs in xss: for x in xs: flat_list.append(x) Here is the corresponding function: def flatten(xss): return [x …
python - How to group dataframe rows into list in pandas groupby ...
2017年12月5日 · I was just googling for some syntax and realised my own notebook was referenced for the solution lol. Thanks for linking this. Just to add, since 'list' is not a series function, you will have to either use it with apply df.groupby('a').apply(list) or use it with agg as part of a dict df.groupby('a').agg({'b':list}). You could also use it with ...
python - Most Pythonic way to iteratively build up a list ... - Stack ...
2014年8月31日 · Create an item M. Create a list L and add M to L. Second, loop through the following: Create a new item by modifying the last item added to L. Add the new item to L. As a simple example, say I want to create a list of lists where the nth list contains the numbers from 1 to n. I could use the following (silly) procedure.
python - How do I fast make a list of 1~100? - Stack Overflow
2015年4月4日 · Depends, if you are using Python 2.X it does but for Python 3.X it produces a range object which should be iterated upon to create a list if you need to. But in any case for all practical purpose extending a range object as a List comprehension is useless and have an unnecessary memory hogging.
python - Pandas DataFrame column to list - Stack Overflow
2014年5月20日 · This is because tst[lookupValue] is a dataframe, and slicing it with [['SomeCol']] asks for a list of columns (that list that happens to have a length of 1), resulting in a dataframe being returned. If you remove the extra set of brackets, as in tst[lookupValue]['SomeCol'] , then you are asking for just that one column rather than a list of ...
Creating a list of integers in Python - Stack Overflow
2021年8月19日 · In python an easy way is: your_list = [] for i in range(10): your_list.append(i) You can also get your for in a single line like so: your_list = [] for i in range(10): your_list.append(i) Don't ever get discouraged by other people's opinions, specially for new learners.
python - Converting Dictionary to List? - Stack Overflow
A list of lists is a valid python list, and a much better interpretation of a "dictionary" than a flat list containing only non-list values. A list of lists is at least capable of showing keys and values still grouped together.