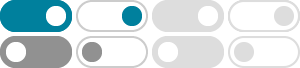
javascript - Why is there no logical XOR? - Stack Overflow
Dec 27, 2010 · Javascript has a bitwise XOR operator : ^ You can use it with booleans and it will give the result as a 0 or 1 (which you can convert back to boolean, e.g. result = !!(op1 ^ op2)). But as John said, it's equivalent to result = (op1 != op2), which is clearer. You can use it as a logical xor. true^true is 0, and false^true is 1.
Bitwise XOR (^) - JavaScript | MDN - MDN Web Docs
Mar 6, 2025 · The bitwise XOR (^) operator returns a number or BigInt whose binary representation has a 1 in each bit position for which the corresponding bits of either but not both operands are 1.
Is there anyway to implement XOR in javascript
Unfortunately, JavaScript does not have a logical XOR operator. You can "emulate" the behaviour of the XOR operator with something like: if( !foo != !bar ) { ... } The linked article discusses a couple of alternative approaches.
JavaScript Bitwise - W3Schools
JavaScript Bitwise XOR. When a bitwise XOR is performed on a pair of bits, it returns 1 if the bits are different:
XOR(^) Bitwise Operator in JavaScript - GeeksforGeeks
Apr 22, 2024 · In JavaScript, the bitwise XOR(^) Operator is used to compare two operands and return a new binary number which is 1 if both the bits in operators are different and 0 if both the bits in operands are the same.
Logical XOR in JavaScript - How To Create
XOR (exclusive OR) is a boolean operator, like && and ||, but with the following logic: It is successful if the expression on either side is true (like ||), but not if both sides are true (like !( x && y )).
Atomics.xor() - JavaScript | MDN - MDN Web Docs
Feb 11, 2025 · The Atomics.xor() static method computes a bitwise XOR with a given value at a given position in the array, and returns the old value at that position. This atomic operation guarantees that no other write happens until the modified value is written back.
JavaScript Bitwise Operators (with Examples) - Programiz
JavaScript Bitwise Operators. Bitwise operators treat its operands as a set of 32-bit binary digits (zeros and ones) and perform actions. However, the result is shown as a decimal value.
JavaScript Bitwise XOR (^) Operator Usage Explained
Nov 11, 2022 · The bitwise XOR operator in JavaScript is a bitwise operator that takes two operands and returns a one in the corresponding bit position if the operands are different, and a zero if they are the same. This operator is typically used for bitwise operations, such as setting or clearing individual bits in a byte or word.
What is ^ in JavaScript - Altcademy Blog
Sep 12, 2023 · The caret symbol (^) in JavaScript is commonly known as the bitwise XOR operator. If that sounds like jargon, don’t worry. Let’s break it down to make it simple.
Bitwise XOR assignment (^=) - JavaScript - RealityRipple
The bitwise XOR assignment operator (^=) uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable.
Mastering the Operations of JavaScript XOR - Itsourcecode.com
Jul 12, 2023 · XOR, short for exclusive OR, is a logical operation that returns true if literally one of the operands is true. In JavaScript, the XOR operation can be completed using the caret symbol “ ^ “. The XOR operator requires two operands and returns a …
Compute the Bitwise XOR of all Numbers in a Range using JavaScript
May 2, 2024 · In JavaScript, the bitwise XOR(^) Operator is used to compare two operands and return a new binary number which is 1 if both the bits in operators are different and 0 if both the bits in operands are the same.
Bitwise XOR - JavaScript - W3cubDocs
Bitwise XOR (^) The ^ operator returns a number or BigInt whose binary representation has a 1 in each bit position for which the corresponding bits of either but not both operands are 1 . Try it
Using the XOR Operator in JavaScript – 5k.io
In JavaScript, the XOR operator (^) allows you to perform a logical exclusive OR operation on two operands. The XOR operator returns true if one of the operands is true and the other operand is false , and it returns false if both operands are true or if both operands are false .
JavaScript Logical Operations - 30 seconds of code
May 7, 2023 · The logical xor (^) operator returns true if exactly one of the operands is true, otherwise it returns false. While JavaScript implements the XOR (^) operator, it's only for bitwise operations. It's not a logical operator, so it doesn't work with booleans.
How to use the bitwise XOR (^) operator in JavaScript
Sep 13, 2022 · If the XOR operator (^ in JS) is applied on two bits, it returns: 1 (true) if they are alternating. 0 (false) if they are the same. So if you run the following snippet, you'll see:
XOR JavaScript – Simplified Guide To Implement XOR In JavaScript
To implement XOR in JavaScript, follow these steps: Declare two variables to hold the values you want to compare. Use the XOR operator (^) to perform the XOR operation on the variables.
Bitwise XOR (^) - JavaScript - RealityRipple
The bitwise XOR operator (^) returns a 1 in each bit position for which the corresponding bits of either but not both operands are 1s.
Bitwise XOR Operator in JavaScript - Delft Stack
Feb 15, 2024 · XOR is a bitwise Boolean operator which only takes true or false operands and returns only true or false. Consequently, JavaScript does not have a logical XOR operator since JavaScript traces its lineage back to the C language, and …
- Some results have been removed