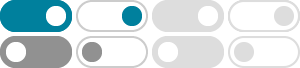
Linear regression with matplotlib / numpy - Stack Overflow
import numpy as np import matplotlib.pyplot as plt x = [1,2,3,4] y = [3,5,7,10] # 10, not 9, so the fit isn't perfect coef = np.polyfit(x,y,1) poly1d_fn = np.poly1d(coef) # poly1d_fn is now a function which takes in x and returns an estimate for y plt.plot(x,y, 'yo', x, poly1d_fn(x), '--k') #'--k'=black dashed line, 'yo' = yellow circle marker ...
python numpy/scipy curve fitting - Stack Overflow
For instance, a linear fit would use a function like. def func(x, a, b): return a*x + b scipy.optimize.curve_fit(func, x, y) will return a numpy array containing two arrays: the first will contain values for a and b that best fit your data, and the second will be the covariance of the optimal fit parameters.
python - fitting data with numpy - Stack Overflow
from numpy.polynomial import Polynomial p = Polynomial.fit(x, y, 4) plt.plot(*p.linspace()) p uses scaled and shifted x values for numerical stability. If you need the usual form of the coefficients, you will need to follow with
numpy - How to find the appropriate linear fit in Python ... - Stack ...
2015年11月12日 · I am trying to find the most appropriate linear fit for a large amount of data that has linear behaviour for most of samples. The data when plotted in the raw form is as shown below: I need the linear fit that encompasses most of the points as shown by the thick orange line in the figure below:
How do I calculate r-squared using Python and Numpy?
2009年5月21日 · From the numpy.polyfit documentation, it is fitting linear regression. Specifically, numpy.polyfit with degree 'd' fits a linear regression with the mean function. E(y|x) = p_d * x**d + p_{d-1} * x **(d-1) + ... + p_1 * x + p_0. So you just need to calculate the R-squared for that fit. The wikipedia page on linear regression gives full details ...
numpy - How to do exponential and logarithmic curve fitting in …
2010年8月8日 · For fitting y = Ae Bx, take the logarithm of both side gives log y = log A + Bx.So fit (log y) against x.. Note that fitting (log y) as if it is linear will emphasize small values of y, causing large deviation for large y.
numpy - How to apply piecewise linear fit in Python ... - Stack …
2015年4月1日 · You are looking for Linear Trees. They are the best method to apply, in a generalized and automated way, a piecewise linear fit (also for multivariate and in classification contexts). Linear Trees differ from Decision Trees because they compute linear approximation (instead of constant ones) fitting simple Linear Models in the leaves.
How to force zero interception in linear regression?
2012年4月3日 · As @AbhranilDas mentioned, just use a linear method. There's no need for a non-linear solver like scipy.optimize.lstsq. Typically, you'd use numpy.polyfit to fit a line to your data, but in this case you'll need to do use numpy.linalg.lstsq directly, as you want to set the intercept to zero. As a quick example:
python - numpy polyfit passing through 0 - Stack Overflow
2015年8月28日 · Import curve_fit from scipy, i.e. from scipy.optimize import curve_fit import matplotlib.pyplot as plt import numpy as np Define the curve fitting function. In your case, def fit_func(x, a, b, c): # Curve fitting function return a * x**3 + b …
Calculating Slopes in Numpy (or Scipy) - Stack Overflow
2012年3月2日 · The linear regression calculation is, in one dimension, a vector calculation. This means we can combine the multiplications on the entire Y matrix, and then vectorize the fits using the axis parameter in numpy.