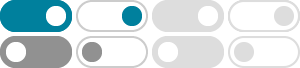
Python, Pandas : write content of DataFrame into text File
2015年7月6日 · Way to get Excel data to text file in tab delimited form. Need to use Pandas as well as xlrd. import pandas as pd import xlrd import os Path="C:\downloads" wb = pd ...
Reading an Excel file in python using pandas - Stack Overflow
2013年6月12日 · import pandas as pd # open the file xlsx = pd.ExcelFile("PATH\FileName.xlsx") # get the first sheet as an object sheet1 = xlsx.parse(0) # get the first column as a list you can loop through # where the is 0 in the code below change to the row or column number you want column = sheet1.icol(0).real # get the first row as a list you can loop ...
Pandas cannot open an Excel (.xlsx) file - Stack Overflow
I had the same problem using the ExcelFile constructor (for a file containing multiple worksheets) instead of the read_excel method. In that case the solution is: In that case the solution is: import pandas xlsx = pandas.ExcelFile('cat.xlsx', engine='openpyxl')
Import CSV file as a Pandas DataFrame - Stack Overflow
To read a CSV file as a pandas DataFrame, you'll need to use pd.read_csv, which has sep=',' as the default.. But this isn't where the story ends; data exists in many different formats and is stored in different ways so you will often need to pass additional parameters to read_csv to ensure your data is read in properly.
How to read file with space separated values in pandas
2018年11月28日 · I try to read the file into pandas. The file has values separated by space, but with different number of spaces I tried: pd.read_csv('file.csv', delimiter=' ') but it doesn't work
Writing a pandas DataFrame to CSV file - Stack Overflow
2019年5月21日 · The below code creates a zip file named compressed_data.zip which has a single file in it named data.csv. df.to_csv('compressed_data.zip', index=False, compression={'method': 'zip', 'archive_name': 'data.csv'}) # read the archived file as a csv pd.read_csv('compressed_data.zip') You can even add to an existing archive; simply pass …
python - How to write to an existing excel file without overwriting ...
2) Now It resize all columns based on cell content width AND all variables will be visible (SEE "resizeColumns") 3) You can handle NaN, if you want that NaN are displayed as NaN or as empty cells (SEE "na_rep") 4) Added "startcol", you can decide to start to write from specific column, oterwise will start from col = 0 ***** """ from openpyxl ...
Python pandas: how to specify data types when reading an Excel …
2015年9月15日 · pd.read_excel('file_name.xlsx', dtype=str) # (or) dtype=object 2) It even supports a dict mapping wherein the keys constitute the column names and values it's respective data type to be set especially when you want to alter the dtype for a subset of all the columns.
Pandas: Looking up the list of sheets in an excel file
Benchmarking on an 8MB XLSX file with nine sheets: %timeit pd.read_excel('foo.xlsx', sheet_name=None, nrows=0).keys() >>> 145 ms ± 50.4 ms per loop (mean ± std. dev. of 7 runs, 10 loops each) %timeit pd.read_excel('foo.xlsx', sheet_name=None).keys() >>> 16.5 s ± 2.04 s per loop (mean ± std. dev. of 7 runs, 1 loop each)
Import multiple CSV files into pandas and concatenate into one …
path = r'C:\DRO\DCL_rawdata_files' # use your path all_files = glob.glob(os.path.join(path, "*.csv")) # advisable to use os.path.join as this makes concatenation OS independent df_from_each_file = (pd.read_csv(f) for f in all_files) concatenated_df = pd.concat(df_from_each_file, ignore_index=True) # doesn't create …