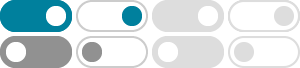
c++ - How to use the priority queue STL for objects? - Stack …
2013年10月23日 · The C++ standard library defines a class template priority_queue, with the following operations: push : Insert an element into the prioity queue. top : Return (without removing it) a highest priority element from the priority queue.
c++ - How to iterate over a priority_queue? - Stack Overflow
C++ priority_queue does not offer a .begin() pointer (like vector would do) that you can use to iterate over it. If you want to iterate over the priority queue to search for whether it contains a value then maybe create a wrapper priority queue and use a hash set to keep track of what you have in the queue.
declaring a priority_queue in c++ with a custom comparator
2015年2月2日 · I'm trying to declare a priority_queue of nodes, using bool Compare(Node a, Node b) as the comparator function (which is outside the node class). What I currently have is: priority_queue<Node,
How to preallocate (reserve) a priority_queue<vector>?
2015年3月24日 · How can I preallocate a std::priority_queue with a container of type std::vector? std::priority_queue<unsigned char, std::vector<unsigned char>> pq; pq.c.reserve(1024); Does not compile because the underlying vector is a protected member. Is it possible to use the constructor of the priority_queue to wrap it around a pre-reserved vector?
stl priority_queue of C++ with struct - Stack Overflow
When priority queue will sort your data then it will need a way to know how to compare among them. You have to specify this by passing a function to priority queue or overloading operator in you custom data class or structure. You can check this answer. This might help you. I have tried to explain multiple ways of using priority queue for ...
Priority Queue of pointers C++ - Stack Overflow
2013年12月19日 · I created a priority queue from vector of node pointers: priority_queue<Node*, vector<Node*>, Comparator > queue; But after some operations I get segmentation fault.
C++ use std::greater () in priority_queue and sort - Stack Overflow
2019年7月5日 · priority_queue<int, vector<int>, greater<int>> minheap; greater<int> is a template argument that specifiers a type and corresponds to the type template parameter class Compare of the priority_queue. template<class T, class Container = vector<T>, class Compare = less<typename Container::value_type>> class priority_queue; In this statement
c++ - Order of elements with the same priority in …
2019年10月2日 · The question is, how those elements are placed in priority_queue? In an order that satisfies the heap property. Which for equal elements is any order. It is importatnt to me that, if any new element comes to priority_queue should be placed after the last element with the same priority. You can achieve that by making the order part of the priority.
Priority_queue functor use C++ - Stack Overflow
2014年10月17日 · Now we can declare our priority_queue which will be based upon elements from the struct and which will be ordered by functor called TheWayILike. priority_queue<SPerson, TheWayILike> or shorter way by using typedef and single name like so: typedef priority_queue<SPerson, TheyWayILike> newNameForPQ;
Initializing and Inserting into a Priority Queue (C++)
2014年2月15日 · I've never used the STL C++ priority queue before and I find the details on the website a little confusing. I want to create a priority queue of Nodes, which I have defined as: struct Node { string data; int weight; Node *left, *right; } I also to insert into the queue in ascending order based on the weight of the nodes.