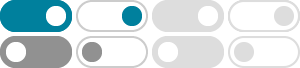
C++ and SDL: How does SDL_Rect work exactly? - Stack Overflow
2010年10月14日 · To dynamically allocate it you'd have to use new otherwise the compiler will interpret your code as a call to a regular function called SDL_Rect that returns a SDL_Rect*. In this case I see no reason to use dynamical allocation; just use the struct initialization syntax (and be careful of the declaration order of the struct's members):
c - Drawing a rectangle with SDL2 - Stack Overflow
2014年2月20日 · Initialize SDL_Window and SDL_Renderer. SDL_Renderer and SDL_Window needs to be set up before you can use them. You already create your window properly, so I won't cover that.
c - Purpose of 'SDL_Rect' structure in SDL 2.0 - Stack Overflow
2019年8月1日 · A SDL_Rect is just a good and easy way of storing the informations of a rectangle. The SDL_Rect struct contains x and y coordinates as well as a width and height. These can be accessed through x, y, w and h in the struct. This can be used to store the dimensions and positions of rectangular textures.
c++ - Comparing two SDL_Rect types - Stack Overflow
2014年6月23日 · I have defined a newRects array of SDL_Rect types to display a grid of rectangles and initialized it. It works fine. SDL_Rect newRects[Max_Rows][Max_Columns]; Now I want to see if in my grid of rectangles, if there is a black rectangle so I define the following function. It returns the matched rect of SDL_Rect type.
c - defining sdl_rect and sdl_mousebuttondown - Stack Overflow
2012年2月27日 · Lets say you create a SDL_Rect structure containing the rectangle you want. When you get the coordinates of a mouse-click it's very simple to compare it with the rectangle coordinates: When you get the coordinates of a mouse-click it's very simple to compare it with the rectangle coordinates:
c - SDL - Blitting an Array of SDL_Rect - Stack Overflow
2016年7月23日 · I built an array of SDL_Rect using malloc and attributed to each member the usual x,y,w (width), and h (height) variables, but the images simply won't show up. Curiously, when I try blitting outside of a for loop by directly referencing the index of …
c++ - SDL render rect from a class - Stack Overflow
2018年5月6日 · I'm trying to render a SDL_Rect in a class, I send the renderer to the "draw" function of my class, and then render it from there. void Tray::draw(SDL_Renderer *renderer){ SDL_RenderFillRect(renderer, &bckRect); } When I put the same code on my main loop. All goes fine, but when I do this from my tray class, It compiles, but doesn't run.
Casting/Changing type to pointer to type (SDL_Rect)
2016年1月19日 · I'm trying to pass a SDL_Rect to SDL_RenderCopy, which takes the adress of a SDL_Rect. I've got the SDL_Rect I'm trying to pass stored in my private class (called "Shot") variabes in the Shot.h file. This is all happening in Shot.cpp. My way of doing it: SDL_RenderCopy(other args..., &Shot::rect) However visual studio complains that
How do I draw multiple rects to the screen in SDL2?
2021年12月17日 · Edit: I think I am having this problem as I thought that SDL_Rect(s) were read and write turns out it is read only. Still don't know what to do now. I am coding in c by the way. Edit: This is what I tried. I don't have concrete code because it doesn't work, however my idea is fundamentally flawed as my thought process was: Make a SDL_Rect array.
SDL - Blit Surface passing float values as rect parameter
2015年5月21日 · You should still represent your objects' coordinates as float, but convert them to int when passing them to your SDL_Rect. Since your SDL_Surfaces will always land perfectly on screen pixels, your graphics should always remain crisp. That being said, to have more precision, I guess you could switch to rendering quads in OpenGL. The hardware ...