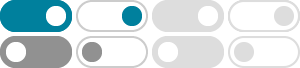
c++ - How to convert vector to set? - Stack Overflow
All of the answers so far have copied a vector to a set. Since you asked to 'convert' a vector to a set, I'll show a more optimized method which moves each element into a set instead of copying each element: std::vector<T> v = /*...*/; std::set<T> s(std::make_move_iterator(v.begin()), std::make_move_iterator(v.end()));
What is the difference between std::set and std::vector?
2011年12月31日 · Of course you could maintain a vector of unique items, but your performance would suffer a lot when you do set-oriented operations. For example, assume that you have a set of 10000 items and a vector of 10000 distinct unordered items. Now suppose that you need to check if a value X is among the values in the set (or among the values in the vector).
Vector of Sets in C++ - Stack Overflow
2012年7月5日 · How to do the vector of sets in C++? I want to have a set for the different levels that are in my code. A set at each level will be holding integer values. The number of these sets should be dynamic depending on the number of levels required ( which is given as input ). For this, I wanted to have a dynamic set structure. How can I achieve this?
How to do the vector of sets in C++? - Stack Overflow
2011年4月8日 · If vector < set< char >> v is exactly what you've got there (I hope you cut and pasted), you've run into one of the annoying little features of C++. Those >> look to you like two closing angle brackets for two templates. They look like a right shift operator to the compiler. Change them to > > with a space in between.
Set of vectors in c++ - Stack Overflow
2014年6月30日 · You're close. You need to pull the vector out of the set iterator. See below. main() { std::set< std::vector<int> > conjunto; std::vector<int> v0 = std::vector<int>(3 ...
c++ - Create set of vectors - Stack Overflow
2019年8月27日 · I am trying to create a set of vectors in C++. I want the vectors [1,2] and [2,1] to be considered equal in the set. So both should not exist in the set. Also a vector can have the same element mul...
c++ - How to set a range of elements in an stl vector to a …
2009年7月17日 · I have a vector of booleans. I need to set its elements from n-th to m-th to true. Is there an elegant way to do this without using a loop? Edit: Tanks to all those who pointed out the problems with using vector<bool>. However, I was looking for a more general solution, like the one given by jalf.
How to change a particular element of a C++ STL vector
So, I created a vector: std::vector<int> vec = {10, 20, 30, 40, 50}; and I ran std::cout << vec[5]; multiple times in my gcc and every time, it displayed 0. On the other hand, when I tried running std::cout << vec.at(5); , I got terminate called after throwing an instance of 'std::out_of_range' what(): vector::_M_range_check: __n (which is 5 ...
C++, copy set to vector - Stack Overflow
In particular, this allows vector to only do one allocation (since it can't determine the distance of set iterators in O(1)), and, if it wasn't defined for vector to zero out each element when constructed, this could be worthwhile to allow the copy to boil down to a memcpy.
Memset on vector C++ - Stack Overflow
2009年11月3日 · If your vector contains POD types, it is safe to use memset on it - the storage of a vector is guaranteed to be contiguous. memset(&vec[0], 0, sizeof(vec[0]) * vec.size()); Edit: Sorry to throw an undefined term at you - POD stands for Plain Old Data, i.e. the types that were available in C and the structures built from them.