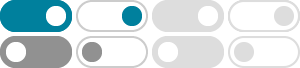
c++ - How do iterate QMap in other QMap - Stack Overflow
2019年3月15日 · You can use QMap::keys() with QMap::value() or QMap::operator[] to iterate over the list to keys and then use keys to get the values. Another solution could be just to get an std::map from QMap::toStdMap() and iterate over it using range-for loop. You might want to look at QMap::uniqueKeys() also depending on your use-case.
c++ - Iterating over a QMap with for - Stack Overflow
2011年12月15日 · Since Qt 5.10, you can wrap QMap::keyValueBegin and QMap::keyValueEnd into a range and then use a range-based for loop to iterate that range. This works without copying the map data and supports both const and rvalue objects.
How do I populate values of a static QMap in C++ Qt?
2011年11月17日 · static QMap<column_t, QString> initColumnNames(); static QMap<column_t, QString> getColumnNames() { static QMap<column_t, QString> COLUMN_NAMES = initColumnNames(); return COLUMN_NAMES; } } This way COLUMN_NAMES will be initialized with the first call of getColumnNames() and you can set the text codec beforehand.
c++ - QMap::contains () VS QMap::find () - Stack Overflow
QMap source code reveals that there is no special code in QMap::contains() method. In some cases you can use QMap::value() or QMap::values() to get value for a key and check if it is correct. These methods (and const operator[] ) will copy the value, although this is probably OK for most Qt types since their underlying data are copied-on-write ...
c++ - How to find specific value in Qmap - Stack Overflow
2014年9月9日 · A map provides quick access based upon the key (the first argument). So, yes, if you want to know if a value exists (the second argument), you would need to iterate over the map values:-
c++ - Sort actual QMap by key - Stack Overflow
2014年11月2日 · I have QMap<QString, MyClass*>. I need to sort it by key using natural comparison. I do: std::map<QString, MyClass*> map = c.toStdMap(); std::sort(map.begin(), map.end(), strnatcmp1<std::pair<QString, MyClass*>>); However, this does not even compile. And even if it did, as far as I understand, it would sort a copy of my original QMap.
qt - Order of items in QMap and QMultiMap - Stack Overflow
2015年12月6日 · From the Qt documentation about QMap::iterator: Unlike QHash, which stores its items in an arbitrary order, QMap stores its items ordered by key. Items that share the same key (because they were inserted using QMap::insertMulti(), or due to a unite()) will appear consecutively, from the most recently to the least recently inserted value.
c++ - QMap::insertMulti or QMultiMap? - Stack Overflow
2015年11月6日 · QMap stores its data in Key order; if order doesn't matter QHash is a faster alternative. QMultiMap<Key, T> This is a convenience subclass of QMap that provides a nice interface for multi-valued maps, i.e. maps where one key can be associated with multiple values. it looks like both can do the job.
c++ - Convert QMap to JSON - Stack Overflow
2017年4月19日 · For your purpose, you are looking for QMAP serialization, see this link: Serialization Qt. Try to set up the constructor with a QByteArray, something like this: QByteArray serializeMap(const QMap<QString, int>& map) { QByteArray buffer; QDataStream stream(&buffer, QIODevice::WriteOnly); out << map; return out; }
QMap and QPair, C++, Qt - Stack Overflow
QMap<int, QString> accessById; QMap<QString, QString> accessByName; I'm searching for a better way, something like this: QMap<QPair<int, QString>, QString> multiAccess; but it can not help me (at least I don't know how to do it), because searching in a …